Rebex SSH Shell
SSH shell, tunneling, telnet, ANSI terminal emulation library for .NET
Download 30-day free trial Buy from $999More .NET libraries
-
Rebex SFTP
SFTP client
-
Rebex SSH Pack
SSH Shell + SFTP + SSH server
-
Rebex Total Pack
All Rebex .NET libraries together
Back to feature list...
Terminal emulation
On this page:
Terminal control (Windows Forms)
TerminalControl
object is a Windows Forms terminal emulation control that makes it very simple to add SSH and telnet terminal emulation capabilities to your application.
// create an instance of SSH, connect and log in
ssh = new Ssh();
ssh.Connect(serverName);
ssh.Login(username, password);
// bind the TerminalControl object (that was added
// to the Form using Visual Studio designer) to the
// SSH channel (creates a SSH shell session)
this.Terminal.Bind(ssh);
' create an instance of SSH, connect and log in
Dim ssh As New Ssh()
ssh.Connect(serverName)
ssh.Login(username, password)
' bind the TerminalControl object (that was added
' to the Form using Visual Studio designer) to the
' SSH channel (creates an SSH shell session)
Me.Terminal.Bind(ssh)
Note: TerminalControl
is only supported on Windows.
Tip: You can bind a terminal to a telnet session or to a Serial Port. How to do it please see Terminal Connecting page.
TerminalControl
shares most features with VirtualTerminal
and they are described below. For an overview of features specific to the control,
see Windows Forms Terminal Control page.

Note: Still too complicated? Try a single-purpose SshTerminalControl
or TelnetTerminalControl
controls first.
These can be configured by the Visual Studio designer
and all you need to do to start an SSH or telnet session is to call the Connect
method.
Those controls are less flexible but easier to start with.
Tip: TerminalControl
has many useful configuration options.
Virtual terminal
VirtualTerminal
is a terminal emulation object that is very similar to TerminalControl
, but there is one major difference - it's not visible.
In fact, it's not even a control. Otherwise, it shares most features with TerminalControl
and is suitable for applications that need to interact with SSH or telnet servers
but don't need to display the actual terminal session.
// create an instance of SSH, connect and log in
var ssh = new Rebex.Net.Ssh();
ssh.Connect(serverName);
ssh.Login(username, password);
// create a new instance of VirtualTerminal
// (creates an SSH shell session)
VirtualTerminal terminal = ssh.StartVirtualTerminal();
// use terminal.Scripting object to achieve the desired action
// ...
' create an instance of SSH, connect and log in
Dim ssh = New Rebex.Net.Ssh()
ssh.Connect(serverName)
ssh.Login(username, password)
' create a new instance of VirtualTerminal
' (creates an SSH shell session)
Dim terminal As VirtualTerminal = ssh.StartVirtualTerminal()
' use terminal.Scripting object to achieve the desired action
' ...
Alternatively, use an existing VirtualTerminal
object for a new SSH shell session instead of creating a new one:
// create an instance of SSH, connect and log in
var ssh = new Rebex.Net.Ssh();
ssh.Connect(serverName);
ssh.Login(username, password);
// create an instance of a invisible terminal
var terminal = new VirtualTerminal(80, 25);
// bind the VirtualTerminal object to the
// SSH channel (creates an SSH shell session)
terminal.Bind(ssh);
// use terminal.Scripting object to achieve the desired action
// ...
' create an instance of SSH, connect and log in
Dim ssh = New Rebex.Net.Ssh()
ssh.Connect(serverName)
ssh.Login(username, password)
' create an instance of a invisible terminal
Dim terminal = New VirtualTerminal(80, 25)
' bind the VirtualTerminal object to the
' SSH channel (creates an SSH shell session)
terminal.Bind(ssh)
' use terminal.Scripting object to achieve the desired action
' ...
Tip: VirtualTerminal
has many useful configuration options.
Tip: If you are mostly interested in the scripting API,
use Ssh.StartScripting
instead of Ssh.StartVirtualTerminal
.
The returned Scripting
object is still based on VirtualTerminal
.
ITerminal interface
ITerminal
interface makes it possible to easily write code that targets both TerminalControl
and VirtualTerminal
objects.
It includes Save
and Bind
methods.
Properties such as Options
, Screen
and Scripting
are available as well.
Custom rendering
Although VirtualTerminal
does not have a visible screen, it's possible to attach a custom renderer with SetCustomScreen
extension method.
A custom renderer simply has to implement the IScreen
interface. Both the method and interface are available in Rebex.TerminalEmulation.Interop
namespace.
Screen API
Both TerminalControl
and VirtualTerminal
provide access to their terminal screen through
Screen
property.
It makes it possible to read and alter the screen content.
Powerful scripting API
Both TerminalControl
and VirtualTerminal
provide powerful scripting capabilities through
provide access to their terminal screen through Scripting
property.
Tip: If the scripting API is all you need, simply get a VirtualTerminal
-based scripting object by calling Ssh.StartScripting
method.
History buffer
Terminal objects provide a 'history buffer', where the output that scrolled away from the current screen is persisted.
The maximum size of the history buffer length is configurable. Use TerminalControl
's HistoryMaxLength
property to change the default value.
VirtualTerminal
doesn't use a history buffer by default, but it can be enabled by calling the
appropriate constructor.
Tip: Several aspects of TerminalControl
's scrolling behavior are configurable.
Palettes
TerminalControl
and VirtualTerminal
make it possible to select one of the predefined color palettes:
// use DOS palette
terminal.Palette = TerminalPalette.Dos;
' use DOS palette
terminal.Palette = TerminalPalette.Dos
Alternatively, you can define a custom palette:
// create a new palette
var palette = new TerminalPalette();
// specify custom colors
palette.SetColor(TerminalColor.Red, Color.Red);
palette.SetColor(TerminalColor.Green, Color.Green);
palette.SetColor(TerminalColor.Yellow, Color.Yellow);
// ...
// use the custom palette
terminal.Palette = palette;
' create a new palette
Dim palette = New TerminalPalette()
' specify custom colors
palette.SetColor(TerminalColor.Red, Color.Red)
palette.SetColor(TerminalColor.Green, Color.Green)
palette.SetColor(TerminalColor.Yellow, Color.Yellow)
' ...
' use the custom palette
terminal.Palette = palette
Note: We support xterm
-style 256-color palettes. However, many terminals can only utilize the first 16 colors.
Tip: For a list of color indices, check out the color schemes blog post.
Color schemes
Some legacy monochrome terminals don't take advantage of color palettes, although they use various visual styles for monochrome text.
TerminalControl
and VirtualTerminal
make it possible to define a custom color scheme, assigning different colors to distinct visual styles:
terminal.Options.ColorScheme = ColorScheme.Custom;
// background
terminal.Options.SetColorIndex(SchemeColorName.Background, TerminalColor.Black);
// normal text
terminal.Options.SetColorIndex(SchemeColorName.Foreground, TerminalColor.LightGray);
// highlighted text
terminal.Options.SetColorIndex(SchemeColorName.Bold, TerminalColor.LightYellow);
// single underline
terminal.Options.SetColorIndex(SchemeColorName.SingleUnderline, TerminalColor.LightRed);
// double underline
terminal.Options.SetColorIndex(SchemeColorName.DoubleUnderline, TerminalColor.LightRed);
terminal.Options.ColorScheme = ColorScheme.[Custom]
' background
terminal.Options.SetColorIndex(SchemeColorName.Background, TerminalColor.Black)
' normal text
terminal.Options.SetColorIndex(SchemeColorName.Foreground, TerminalColor.LightGray)
' highlighted text
terminal.Options.SetColorIndex(SchemeColorName.Bold, TerminalColor.LightYellow)
' single underline
terminal.Options.SetColorIndex(SchemeColorName.SingleUnderline, TerminalColor.LightRed)
' double underline
terminal.Options.SetColorIndex(SchemeColorName.DoubleUnderline, TerminalColor.LightRed)
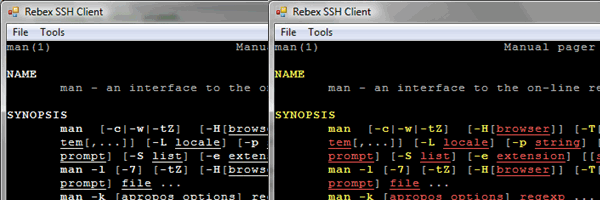
Tip: For a monochrome application, custom styles provide a nice visual enhancement - see the color schemes blogpost for details and a list of color indices.
Note: Color schemes work with color-capable terminals as well. They even make it possible to turn a color terminal
into a monochrome one simply by setting TerminalOption.ColorScheme
property to ColorScheme.Monochrome
.
Cursor styles
TerminalControl
supports three different cursor styles:

// use I-Beam cursor style ( | )
terminal.CursorStyle = CursorStyle.Beam;
' use I-Beam cursor style ( | )
terminal.CursorStyle = CursorStyle.Beam
Fonts
Apart from color palettes and schemes, the appearance of the the terminal depends in large part on the selected font.
To specify a font, use TerminalFont
property:
terminal.TerminalFont = new TerminalFont("Courier New", 10);
terminal.TerminalFont = New TerminalFont("Courier New", 10)
The font specified should be a monospace font whose characters are of constant width. Variable-width fonts are supported as well, but will most likely not look very good.

Note: In addition to Windows fonts, ITerminal
supports legacy raw DOS fonts. Use TerminalFont.FromDosFont
method to load them.
Line drawings characters in all fonts
To ensure the best viewing experience, our terminal controls have built-in drawing routines for lots of special characters. You won't see any gaps in lines composed of various line-drawing characters.

Settings and options
TerminalControl
and VirtualTerminal
classes provide many options to make them compatible with lots of different terminals.
For more information, check out the documentation for the TerminalOptions class
or the Windows Forms Terminal Client sample.
// create a virtual terminal object
VirtualTerminal terminal = new VirtualTerminal(80, 25);
// initialize the options class and set some options
TerminalOptions options = new TerminalOptions();
options.TerminalName = "xterm";
options.TerminalType = TerminalType.Ansi;
options.FunctionKeysMode = FunctionKeysMode.Linux;
options.Encoding = System.Text.Encoding.UTF8;
// assign the options
terminal.Options = options;
' create a virtual terminal object
Dim terminal As New VirtualTerminal(80, 25)
' initialize the options class and set some options
Dim options As New TerminalOptions()
options.TerminalName = "xterm"
options.TerminalType = TerminalType.Ansi
options.FunctionKeysMode = FunctionKeysMode.Linux
options.Encoding = System.Text.Encoding.UTF8
' assign the options
terminal.Options = options
Remote exec
It's possible to start a terminal by launching a remote command instead of a shell. When the command ends, the terminal session ends as well. This is very useful if you need to ensure that the remote command won't suddenly fail and get back to shell without notice.
// instruct the terminal to start
// a command-line FTP client instead of shell
// on a remote server when starting a session
terminal.Options.RemoteCommand = "ftp test.rebex.net";
// bind the terminal to an Ssh object,
// which starts the remote command instead of shell
terminal.Bind(ssh);
' instruct the terminal to start
' a command-line FTP client instead of shell
' on a remote server when starting a session
terminal.Options.RemoteCommand = "ftp test.rebex.net"
' bind the terminal to an Ssh object,
' which starts the remote command instead of shell
terminal.Bind(ssh)
Back to feature list...